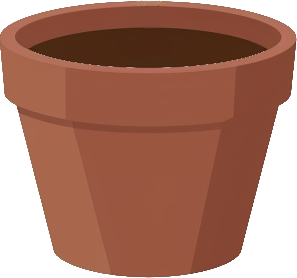
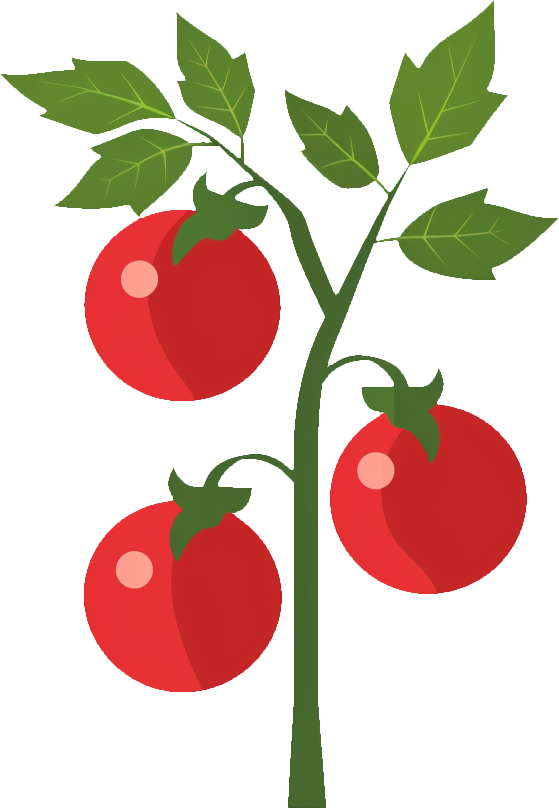
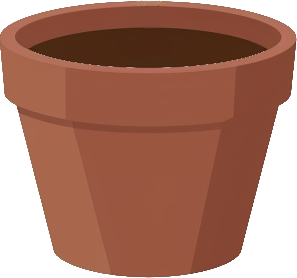
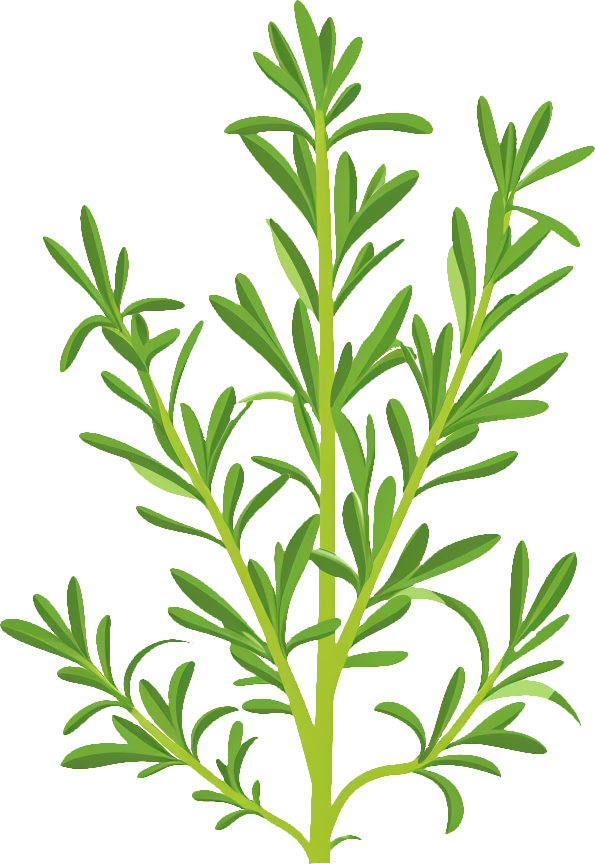
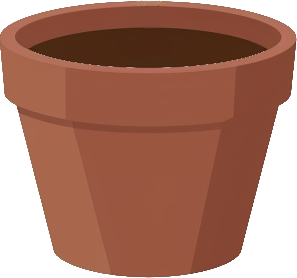
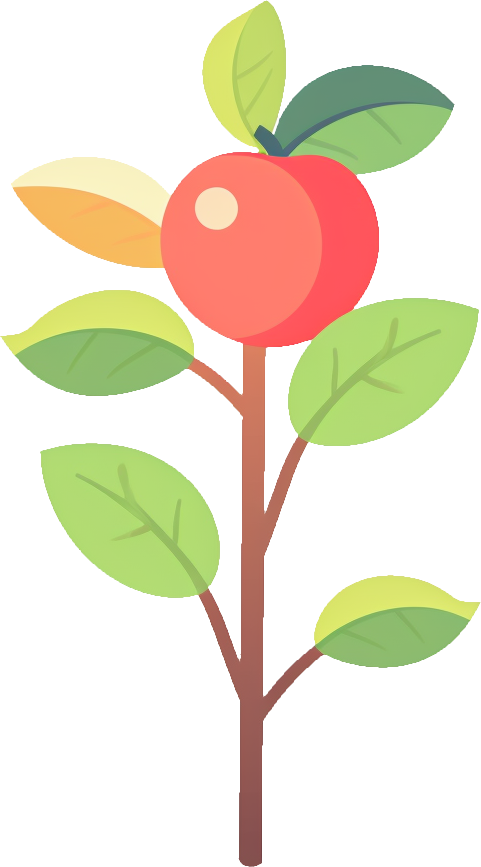
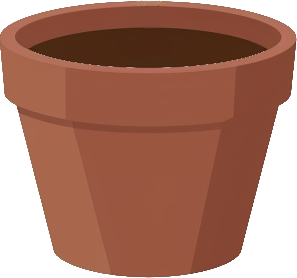
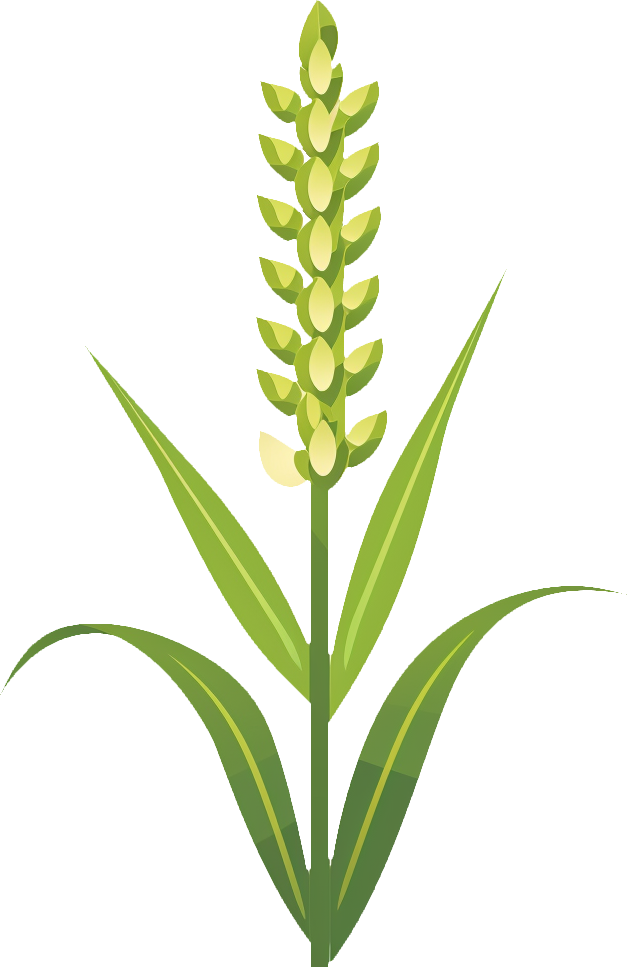
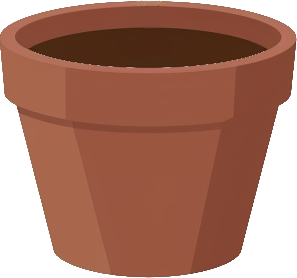
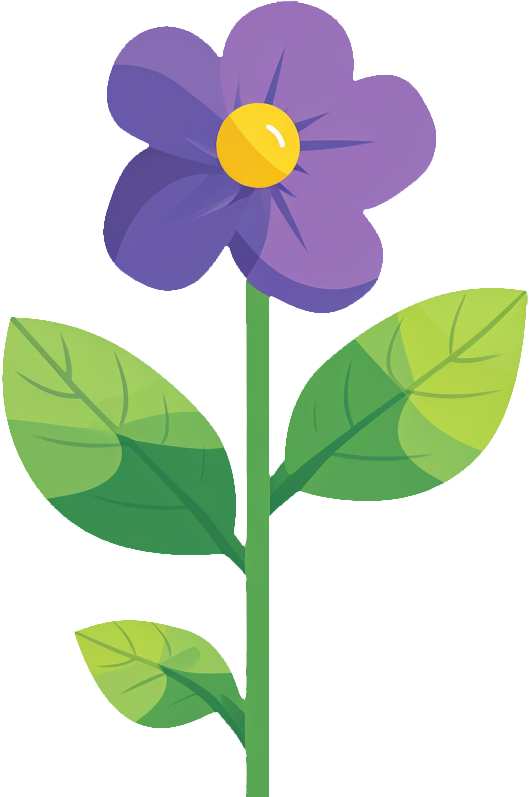
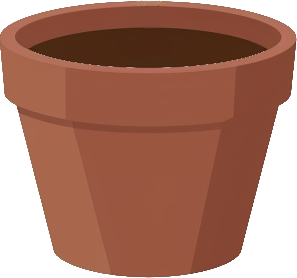
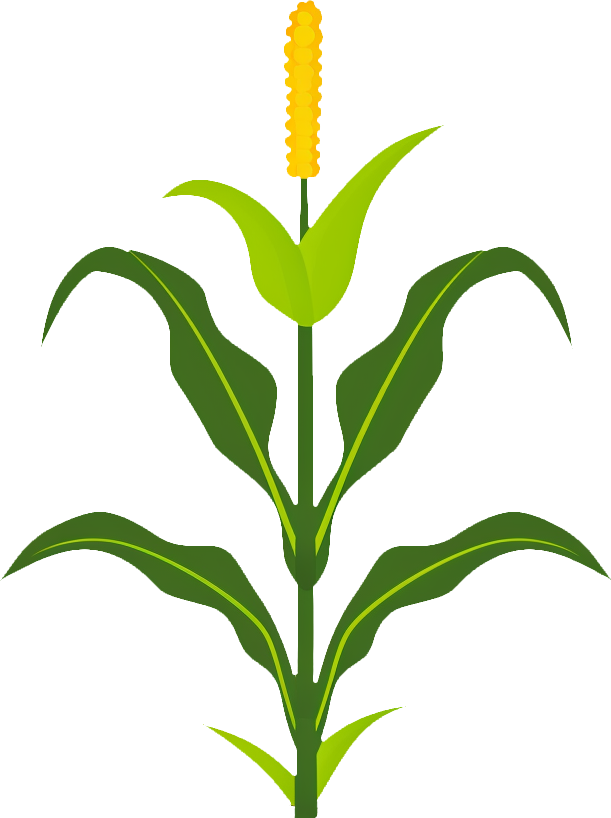
- Garden Text Representation
let garden = [
"Tomato 'Roma VF1'", "Rosemary",
"Apple 'Gala Must 696'",
"Wheat 'Norin 10'", "Primrose",
"Corn 'Pioneer 3751'"
];
- Code Editor
- Log
for (const plant of garden) {
if (plant.match(//i) {
fertilize(plant);
}
}
Lesson task •
Fertilize plants witch name starts with "A", "B" or "C" AND has at least one digit between 0 and 5 or between 7 and 9.
Single character from a custom symbol class
[...] again
Matches single character from the specified range of symbolsRanges can be specified not only between digits, but also between letters:
console.log(!!"a".match(/[a-z]/)) // true
console.log(!!"z".match(/[a-z]/)) // true
console.log(!!"A".match(/[a-z]/)) // false
console.log(!!"Z".match(/[a-z]/)) // false
console.log(!!"a".match(/[A-Z]/)) // false
console.log(!!"z".match(/[A-Z]/)) // false
console.log(!!"A".match(/[A-Z]/)) // true
console.log(!!"Z".match(/[A-Z]/)) // true
You can combine ranges from lowercase to uppercase letters into one range:
console.log(!!"a".match(/[A-z]/)) // true
console.log(!!"z".match(/[A-z]/)) // true
console.log(!!"A".match(/[A-z]/)) // true
console.log(!!"Z".match(/[A-z]/)) // true
But be careful determining case-agnostic range: the range should start with uppercase letter, not reverso: /[a-Z]/ throws an error. This is because under the hood the regular expressions uses ASCII table to determine which symbols are included in the range: it finds the ASCII codes of the start of range and the end of range symbols and all the symbols with codes in between are included in the range. "Z" has lower ASCII code than "a", so the the resulting range /[a-Z]/ is empty.
Other consequence is that /[A-z]/ among the letters in all the cases also includes some special symbols that lies in ASCII table between "A" and "z":
console.log(!!"[".match(/[A-z]/)) // true
console.log(!!"\".match(/[A-z]/)) // true
console.log(!!"]".match(/[A-z]/)) // true
console.log(!!"^".match(/[A-z]/)) // true
console.log(!!"_".match(/[A-z]/)) // true
console.log(!!"`".match(/[A-z]/)) // true
If you want to restrict the range with only latins without special symbols you probably should use combination of two ranges "cutting" off the special symbols in between:
console.log(!!"a".match(/[A-Za-z]/)) // true
console.log(!!"z".match(/[A-Za-z]/)) // true
console.log(!!"A".match(/[A-Za-z]/)) // true
console.log(!!"Z".match(/[A-Za-z]/)) // true
console.log(!!"[".match(/[A-Za-z]/)) // false
console.log(!!"\".match(/[A-Za-z]/)) // false
console.log(!!"]".match(/[A-Za-z]/)) // false
console.log(!!"^".match(/[A-Za-z]/)) // false
console.log(!!"_".match(/[A-Za-z]/)) // false
console.log(!!"`".match(/[A-Za-z]/)) // false
Keeping in ming the underlying mechanics of the symbol classes ranges, we can define ranges of special symbols:
console.log(!!"!".match(/[!-/]/)) // true
console.log(!!""".match(/[!-/]/)) // true
console.log(!!"#".match(/[!-/]/)) // true
console.log(!!"$".match(/[!-/]/)) // true
console.log(!!"%".match(/[!-/]/)) // true
console.log(!!"&".match(/[!-/]/)) // true
console.log(!!"'".match(/[!-/]/)) // true
console.log(!!"(".match(/[!-/]/)) // true
console.log(!!")".match(/[!-/]/)) // true
console.log(!!"*".match(/[!-/]/)) // true
console.log(!!"+".match(/[!-/]/)) // true
console.log(!!",".match(/[!-/]/)) // true
console.log(!!"-".match(/[!-/]/)) // true
console.log(!!".".match(/[!-/]/)) // true
console.log(!!"/".match(/[!-/]/)) // true
Now we can craft ourselves some of the predefined symbol classes from the previous lessons:
- \w equals to [A-Za-z0-9_]
- \s equals to [ \t\n\r\f]
- \d equals to [0-9]