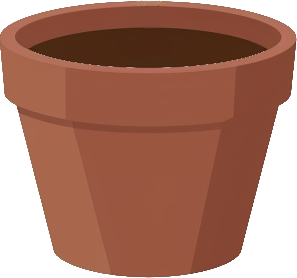
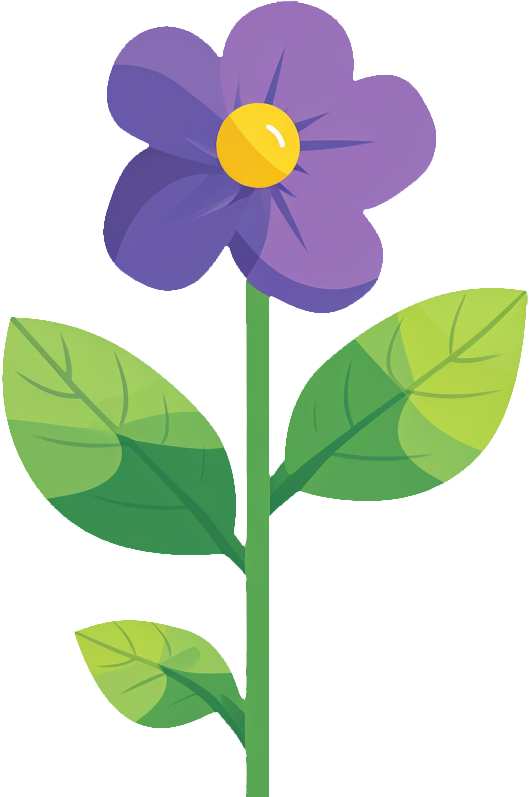
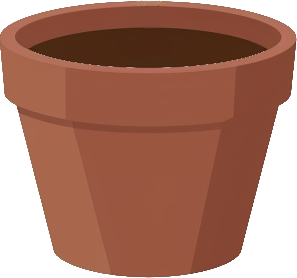
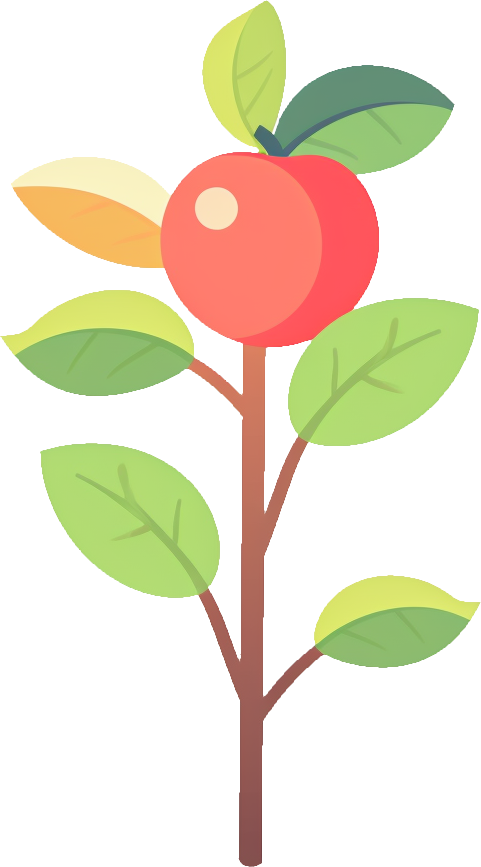
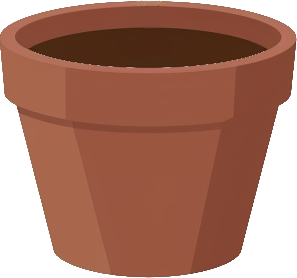
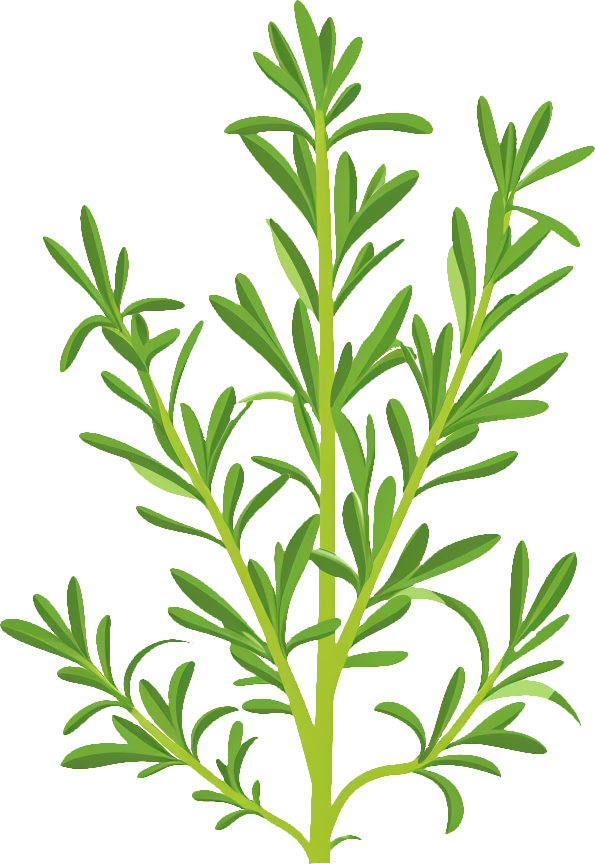
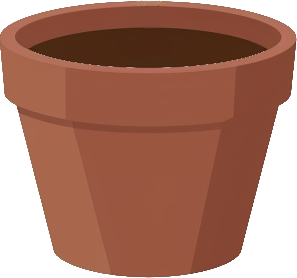
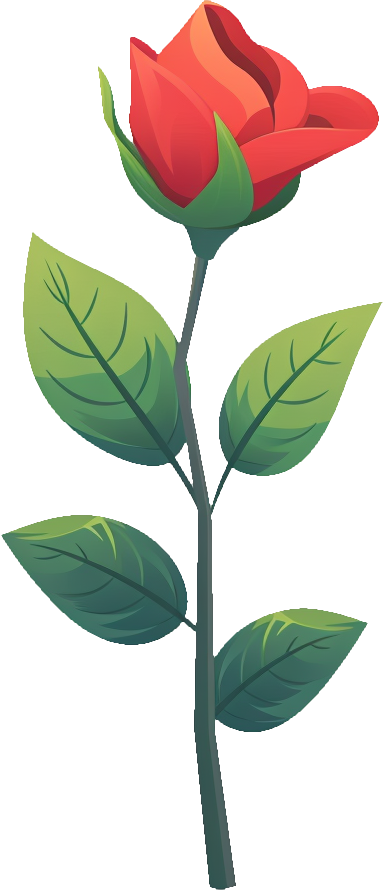
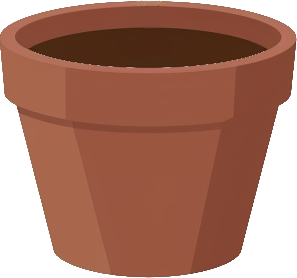
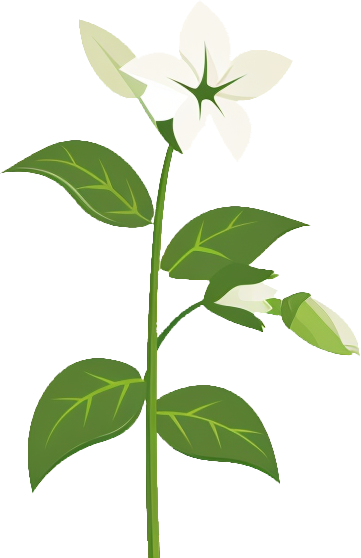
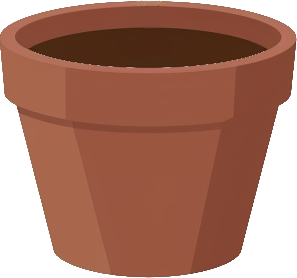
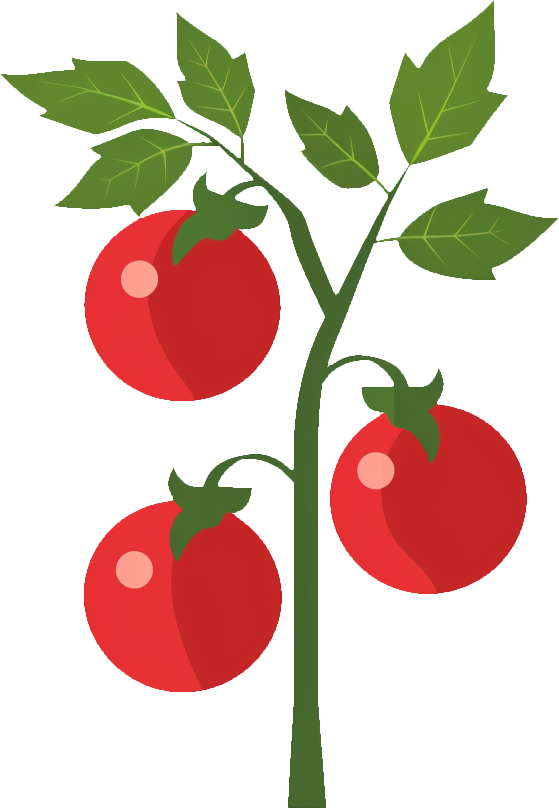
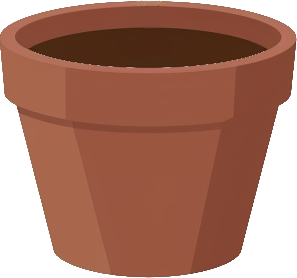
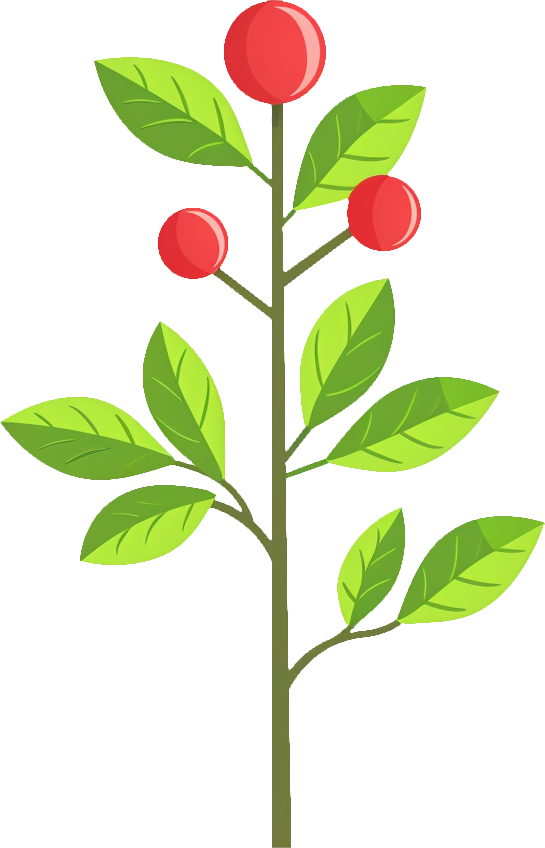
- Garden Text Representation
let garden = [
"Primrose", "Apple 'Gala Must 696'",
"Rosemary", "Rose-of-Sharon",
"Snow-in-Summer", "Tomato 'Roma VF1'",
"Roseberry"
];
- Code Editor
- Log
for (const plant of garden) {
if (plant.match(//i) {
cut(plant);
}
}
Lesson task •
Cut plants that has one or more "'" or "-" in its name
Single character from a custom symbol class
[...]
Matches single character from the specified range of symbolsIn the previous lessons we encountered several symbol classes: \w for word characters only, \s for whitespace characters only, \d for digit characters only, and their opposites. In regular expressions we are not limited by these classes but can create our own classes enumerating allowed symbols inside [ and ]. For example, /[:;]/ will match single ":" or single ";":
console.log(!!":".match(/[:;]/)) // true
console.log(!!";".match(/[:;]/)) // true
console.log(!!"4".match(/[:;]/)) // false
console.log(!!"f".match(/[:;]/)) // false
console.log(!!":::".match(/^[:;]$/)) // false
Please note, that symbols forming a class are enumerated without commas.
Among enumerating symbols forming a class, we can define a range of symbols: /[0-4]/ will match any single digit from the range from 0 to 4:
console.log(!!"0".match(/[0-4]/)) // true
console.log(!!"1".match(/[0-4]/)) // true
console.log(!!"2".match(/[0-4]/)) // true
console.log(!!"3".match(/[0-4]/)) // true
console.log(!!"4".match(/[0-4]/)) // true
console.log(!!"5".match(/[0-4]/)) // false
We can also combine multiple ranges at one time:
console.log(!!"0".match(/[0-46-9]/)) // true
console.log(!!"1".match(/[0-46-9]/)) // true
console.log(!!"2".match(/[0-46-9]/)) // true
console.log(!!"3".match(/[0-46-9]/)) // true
console.log(!!"4".match(/[0-46-9]/)) // true
console.log(!!"5".match(/[0-46-9]/)) // false
console.log(!!"6".match(/[0-46-9]/)) // true
console.log(!!"7".match(/[0-46-9]/)) // true
console.log(!!"8".match(/[0-46-9]/)) // true
console.log(!!"9".match(/[0-46-9]/)) // true
Worth noting that in the regexp above "-" is not included in the range:
console.log(!!"-".match(/[0-46-9]/)) // false
If for some reason, among the digits from 0 to 4 and from 6 to 9, you need to match "-", you have to specify it at the beginning or at the end of the symbol class. When used not at the beginning or at the end, "-" forms a range:
console.log(!!"-".match(/[-0-46-9]/)) // true
console.log(!!"-".match(/[0-46-9-]/)) // true
console.log(!!"0".match(/[0-46-9-]/)) // true
console.log(!!"4".match(/[0-46-9-]/)) // true
console.log(!!"5".match(/[0-46-9-]/)) // false
console.log(!!"6".match(/[0-46-9-]/)) // true
console.log(!!"9".match(/[0-46-9-]/)) // true
There is no need to escape special symbols when enumerating them except backslash and square brackets itself:
console.log(!!".".match(/[.^$\\/*+?\[\]]/)) // true
console.log(!!"^".match(/[.^$\\/*+?\[\]]/)) // true
console.log(!!"$".match(/[.^$\\/*+?\[\]]/)) // true
console.log(!!"\".match(/[.^$\\/*+?\[\]]/)) // true
console.log(!!"*".match(/[.^$\\/*+?\[\]]/)) // true
console.log(!!"+".match(/[.^$\\/*+?\[\]]/)) // true
console.log(!!"?".match(/[.^$\\/*+?\[\]]/)) // true
console.log(!!"[".match(/[.^$\\/*+?\[\]]/)) // true
console.log(!!"]".match(/[.^$\\/*+?\[\]]/)) // true
You can also use symbol class tokens from the previous lessons inside the custom symbol class:
console.log(!!"0".match(/[\d]/)) // true
console.log(!!"1".match(/[\d]/)) // true
console.log(!!"2".match(/[\d]/)) // true
console.log(!!"3".match(/[\d]/)) // true
console.log(!!"4".match(/[\d]/)) // true
console.log(!!"5".match(/[\d]/)) // true
console.log(!!"6".match(/[\d]/)) // true
console.log(!!"7".match(/[\d]/)) // true
console.log(!!"8".match(/[\d]/)) // true
console.log(!!"9".match(/[\d]/)) // true
As usual, if you need to match "[" or "]" literally, consider escaping it with the backslash:
console.log(!!"[".match(/\[/)) // true
console.log(!!"]".match(/\]/)) // true