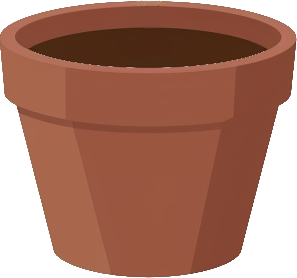
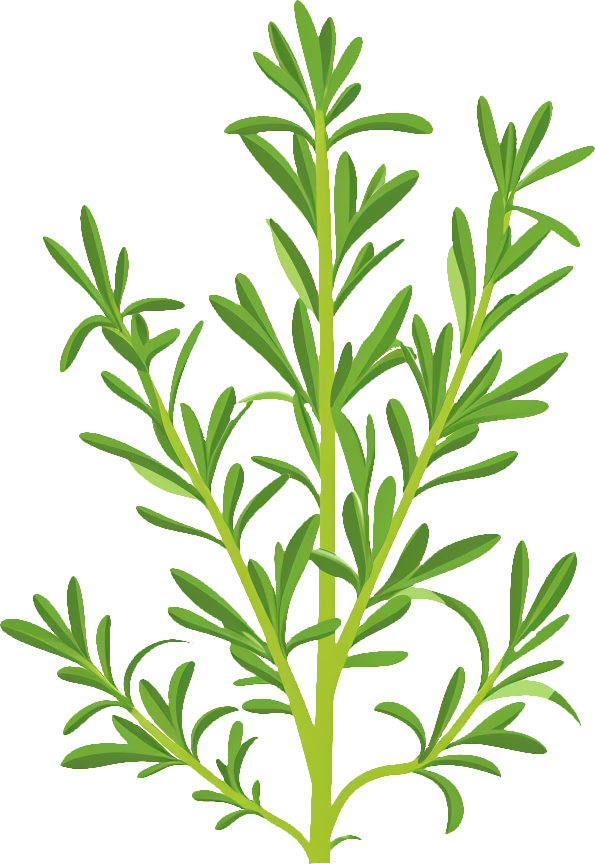
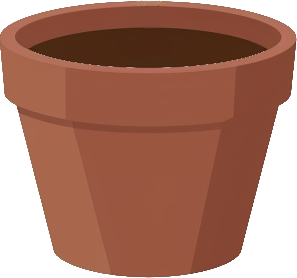
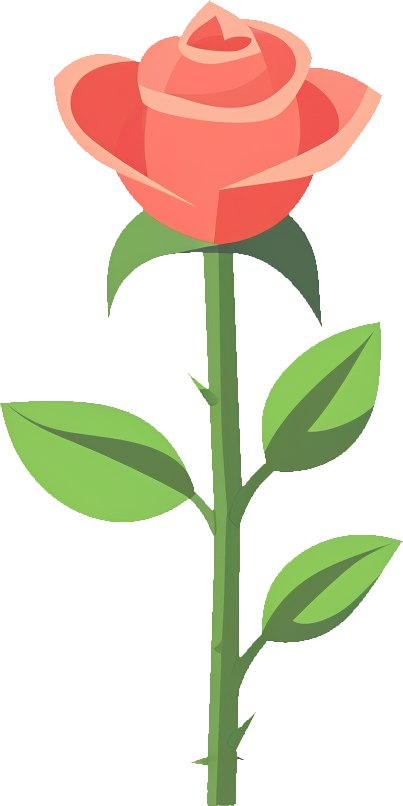
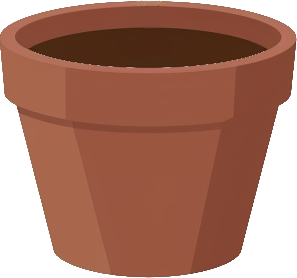
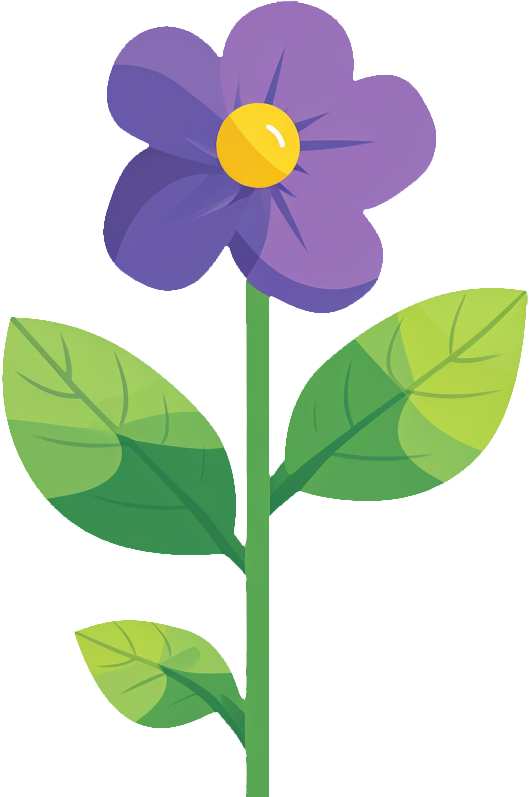
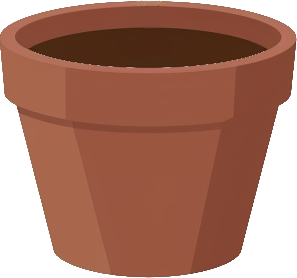
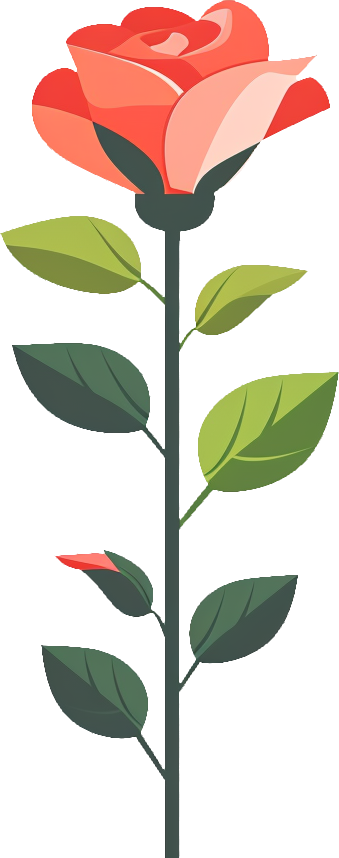
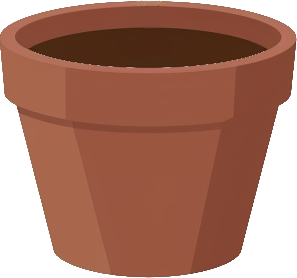
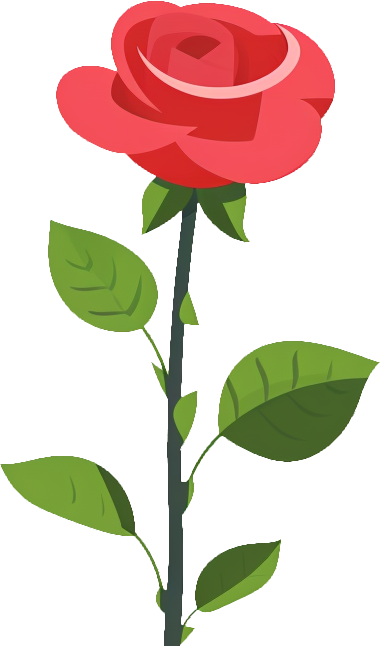
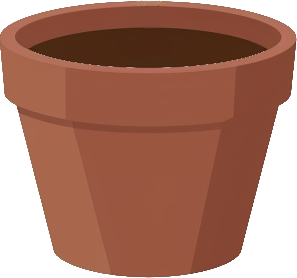
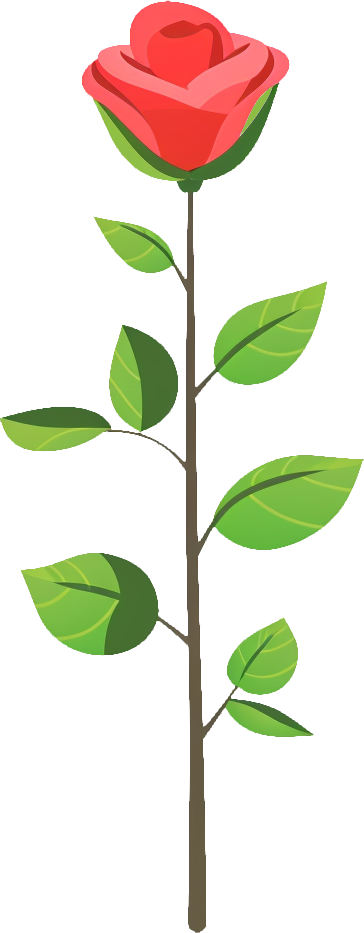
- Garden Text Representation
let garden = [
"Rosemary", "Rose 'Iceberg'",
"Primrose",
"Rose 'Double Delight'", "Rose",
"Rose 'Queen Elizabeth'"
];
- Code Editor
- Log
garden = garden
.map(plant =>
plant.replace(//i, "$1")
)
);
Lesson task •
Remove all the cultivars from the names of the plants
Capturing group
(...)
Saves a part of matched string into a local variable that can be used later in a replacerWith regular expressions you can not only replace one text with another, but you can dynamically define replacer text based on the original text. If you cover a part of the regular expression with round brackets, you can address it in the replacer as "$1":
console.log("foo 42 bar".replace(/^\w+ (.+) \w+/i, "the number in between is $1")) // the number in between is 42
console.log("foo 42".replace(/^\w+ (\d+)/i, "the number after foo is $1")) // the number after foo is 42
You are not restricted with only one capturing group: you can have as much as 10'000 of capturing groups in one regular expression! Each of them can be than addressed in a replacer with "$2", "$3", ..., "$10000":
console.log("Isaac Asimov".replace(/(\w+) (\w+)/i, "$2, $1")) // Asimov, Isaac
console.log("4 8 15 16 23 42".replace(/(\d+) (\d+) (\d+) (\d+) (\d+) (\d+)/i, "$6 $5 $4 $3 $2 $1")) // 42 23 16 15 8 4
Another use-case for capturing group - applying a quantifier to the part of the regular expression wrapped in the brackets:
console.log(!!"foo".match(/(foo\s*){3,}/i)) // false
console.log(!!"foo foo".match(/(foo\s*){3,}/i)) // false
console.log(!!"foo foo foo".match(/(foo\s*){3,}/i)) // true
But there is a more performant way to grouping repetitive parts of the regular expression. We will cover it later.
If you need to match "(" or ")" literally, consider escaping it with the backslash:
console.log(!!"(".match(/\(/)) // true
console.log(!!")".match(/\)/)) // true