RegexpGarden
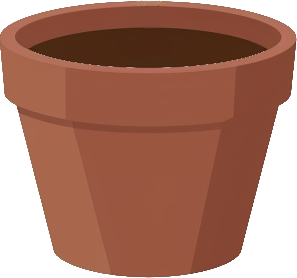
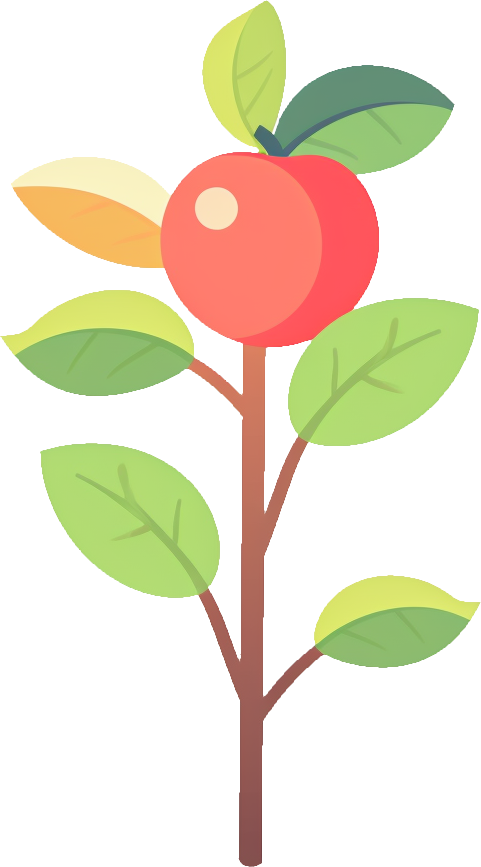
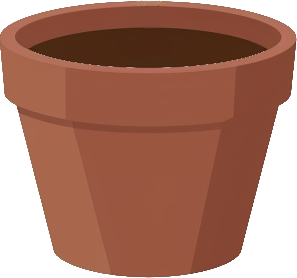
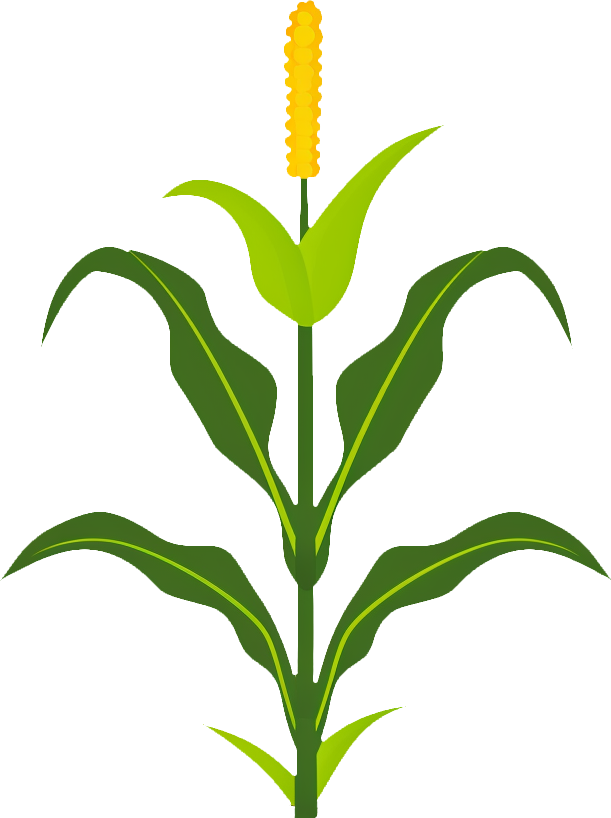
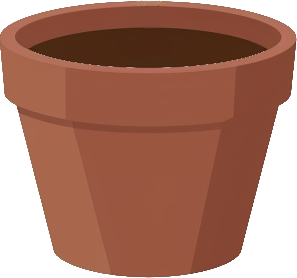
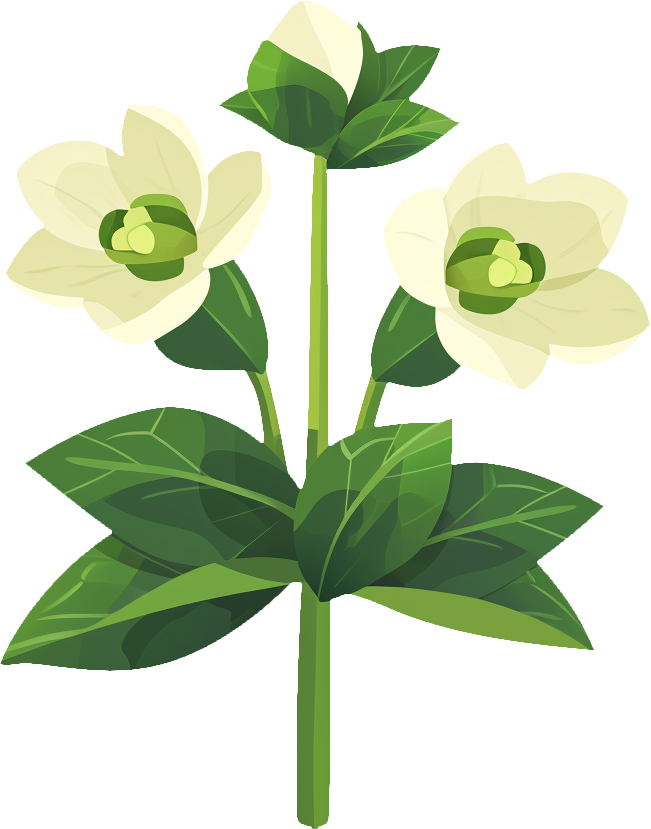
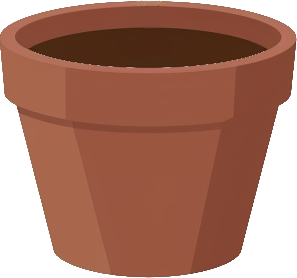
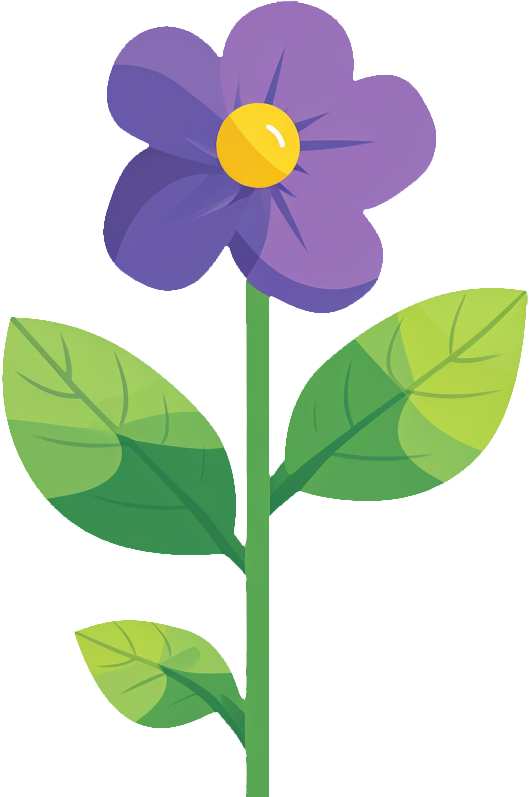
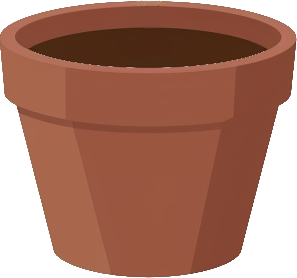
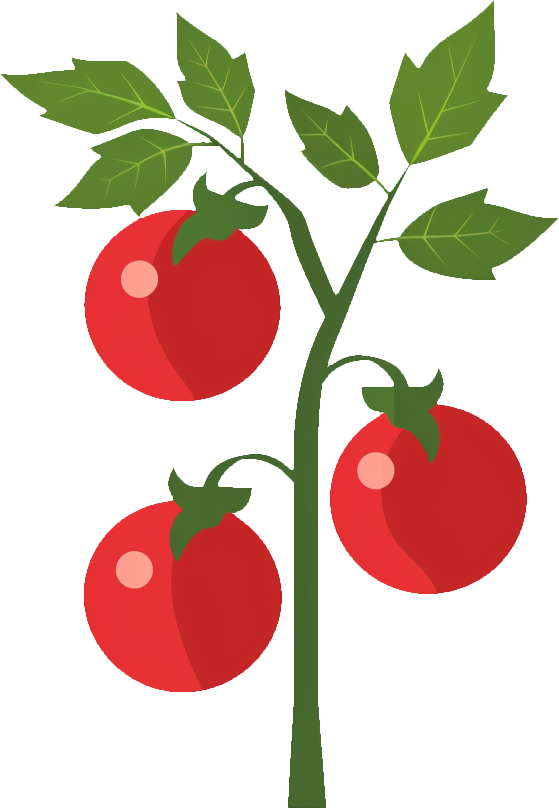
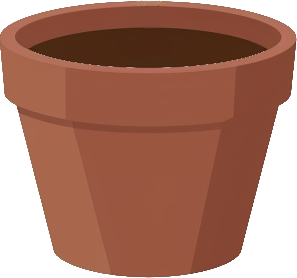
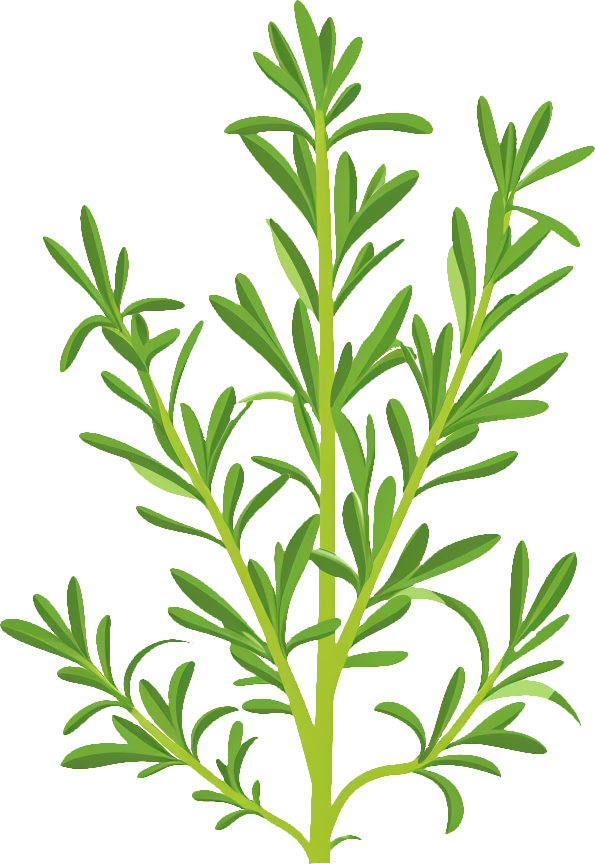
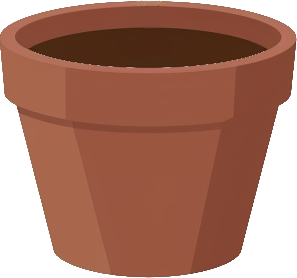
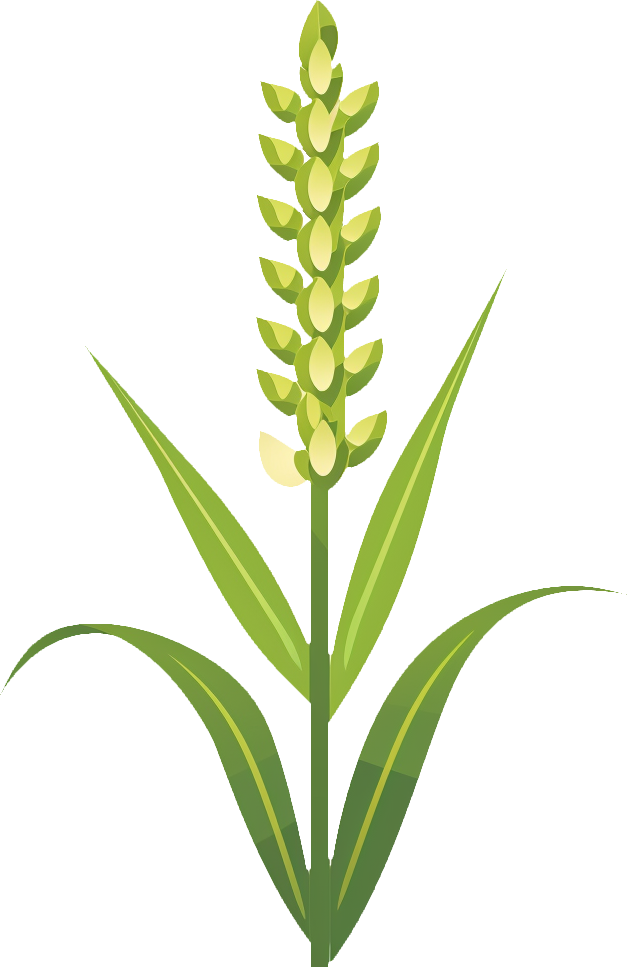
- Garden Text Representation
1234567
let garden = [
"Apple 'Gala Must 696'",
"Corn 'Pioneer 3751'",
"Christmas Rose", "Primrose",
"Tomato 'Roma VF1'", "Rosemary",
"Wheat 'Norin 10'"
];
- Code Editor
- Log
123456
for (const plant of garden) {
if (plant.match(//i) {
fertilize(plant);
}
}
Lesson task •
Fertilize plants which names consist of only word characters
Zero or more times
*
Repeats the previous token zero or more timesIn the previous lessons we've wrote regular expressions where one token match exactly one symbol. Let's say we want to write an expression to match all the strings starting with "foo" and ending with "bar" with any two word characters in between. We would write something like this:
1
console.log(!!"foo42bar".match(/^foo\w\wbar$/)) // true
But what if we don't know in advance how many word characters will be between "foo" and "bar"? What if there could be zero or any word characters in the middle? Our previous expression would fail there:
123
console.log(!!"foo4bar".match(/^foo\w\wbar$/)) // false
console.log(!!"foo423bar".match(/^foo\w\wbar$/)) // false
console.log(!!"foobar".match(/^foo\w\wbar$/)) // false
This is where * would help us:
123
console.log(!!"foo42bar".match(/^foo\w*bar$/)) // true
console.log(!!"foo4bar".match(/^foo\w*bar$/)) // true
console.log(!!"foobar".match(/^foo\w*bar$/)) // true
If you want to match "*" literally, escape it with backslash:
1
console.log(!!"*".match(/\*/)) // true